A Comprehensive Guide to Mastering the Popular JavaScript Library
React has become a crucial technology in the world of web development. In this article, we will dive deep into React and explore its core concepts, benefits, and best practices. Whether you're a beginner or an experienced developer looking to enhance your skills, this comprehensive guide will help you understand React and harness its power to create dynamic and efficient web applications.
React.js offers a wide-range of capabilities that empower development teams to efficiently manage and innovate upon their source code. Whether it's crafting complex user interfaces or architecting scalable applications, React.js provides the tools and flexibility necessary for teams to tackle diverse challenges effectively while maintaining code integrity and fostering collaboration within the development team.
React has revolutionized the way we build user interfaces in web applications. By understanding its core concepts and implementing best practices, developers can create robust and scalable applications. This guide has equipped you with the knowledge and tools to take your React skills to the next level. React today and unlock its full potential!
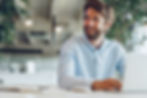
History of React
React.js was created by Jordan Walke, a software engineer at Facebook. The project started in 2011 and was initially developed to address the company's need for a flexible and efficient user interface framework for building complex web applications.
At the time, the web development landscape was dominated by frameworks such as AngularJS and Ember.js, which were built around the concept of two-way data binding. However, the Facebook team found that these frameworks were not well-suited for building large-scale applications, as the constant and automatic synchronization of data made it difficult to reason about the state of the application.
Motivated by the desire to improve the performance and maintainability of their user interfaces, Walke started to explore alternative approaches.
What is React?
React.js is a popular JavaScript library used for building user interfaces in web applications. Its main purpose is to create reusable UI components that can efficiently render and update the user interface based on changes in data. React allows developers to create interactive and dynamic web applications by using a component-based architecture. It uses a virtual DOM (Document Object Model) to efficiently manage UI updates and optimize performance. React.js is widely used in modern web development due to its simplicity, flexibility, and large community support.
React's component-based architecture is one of its core features. It allows you to build reusable UI elements called components. Components encapsulate their own state and logic, making them self-contained and easy to manage. This modular approach promotes code reusability and maintainability.
The virtual DOM, on the other hand, is a representation of the actual DOM in memory. It is a lightweight copy of the real DOM. React uses the virtual DOM to efficiently update only the necessary parts of the UI when changes occur. Instead of updating the entire DOM, React compares the virtual DOM with the real DOM, identifies the differences, and applies only those changes.
Today, React.js is widely used by companies of various sizes. Some of the largest and most well-known companies that utilize React.js include Facebook, Instagram, Netflix, Airbnb, WhatsApp, Uber, Pinterest, Dropbox, Slack, Reddit.
These companies, among many others, have chosen React.js for its flexibility, performance, and robustness in building scalable and interactive web applications. React.js has become a popular choice among developers, making it a valuable tool for both small startups and large enterprises.
Modernizing React: Functional Components and Hooks
Older versions of React primarily relied on class components and lifecycle methods for building user interfaces. These class components allowed developers to encapsulate state and behavior within individual components, making it easier to manage complex UI logic. However, as React evolved, it introduced functional components and hooks, fundamentally changing how developers approach building applications.
In modern React, functional components have become the preferred way of defining UI elements. Functional components are simpler, more concise, and easier to understand compared to class components. They promote a functional programming style, where components are treated as pure functions that take props as input and return JSX elements as output. This functional approach makes code more predictable and easier to test since it reduces the amount of shared mutable state.
One of the key reasons for using the functional approach in modern React is the introduction of hooks. Hooks are functions that enable functional components to use state and other React features without needing to write a class. With hooks like useState, useEffect, useContext, etc., functional components gain the ability to manage state, perform side effects, and access context within a component. This simplifies component logic and encourages the composition of smaller, reusable functions.
Functional components offer performance benefits over class components. Since functional components are essentially just functions, they have less overhead compared to class components, resulting in faster rendering times. Additionally, functional components promote better code organization and readability by encouraging the separation of concerns and reducing boilerplate code.
It's important to note that the older class-based approach in React is not deprecated and is still fully supported. Many existing codebases and libraries still use class components, and they continue to function perfectly well. While the functional approach with hooks has become increasingly popular for its simplicity and flexibility, there are situations where class components may still be the preferred choice, especially in legacy projects or scenarios where specific lifecycle methods are necessary. Both approaches have their strengths and can coexist in the React ecosystem, allowing developers to choose the best tool for the job based on their project requirements and personal preferences.
In this article, we'll look at two ways of writing React components: the older class-based approach and the newer functional approach with hooks. By comparing these methods, we'll see how React has evolved to offer a more concise and efficient way of building user interfaces.
Core Concepts of React
React.js lifecycles are essential to understanding how components behave throughout their existence within an application. These lifecycles consist of various methods that allow developers to execute code at specific points during a component's lifecycle.
Mounting phase
constructor: The constructor method is called when a component is being initialized.
render: The render method is responsible for displaying the component's content.
componentDidMount: This method is called right after the component has been rendered to the DOM.
Updating phase:
shouldComponentUpdate: This method determines whether the component should be re-rendered or not.
render: The render method is called again if the component needs to be updated.
componentDidUpdate: This method is called after the component has been updated in the DOM.
Unmounting phase
componentWillUnmount: This method is called right before the component is removed from the DOM.
You can use the code snippet below to confirm the execution order of those methods.
import React, { Component } from 'react';
class LifecycleComponent extends Component {
constructor(props) {
super(props);
console.log('Constructor called');
}
componentDidMount() {
console.log('Component did mount');
}
componentDidUpdate() {
console.log('Component did update');
}
componentWillUnmount() {
console.log('Component will unmount');
}
render() {
console.log('Render called');
return (
<div>
<h1>Lifecycle Component</h1>
</div>
);
}
}
export default LifecycleComponent;
Below, you can find an alternative version of the component using React hooks. This version utilizes the useEffect hook to handle component lifecycle events. The useEffect hook with an empty dependency array mimics the componentDidMount lifecycle method, while the second useEffect hook without dependencies simulates the componentDidUpdate method. Additionally, the cleanup function within the first useEffect hook serves as the equivalent of the componentWillUnmount lifecycle method. By leveraging hooks, we achieve similar functionality in a more concise and functional manner.
import React, { useEffect } from 'react';
const LifecycleComponent = () => {
useEffect(() => {
console.log('Component did mount');
return () => {
console.log('Component will unmount');
};
}, []);
useEffect(() => {
console.log('Component did update');
});
console.log('Render called');
return (
<div>
<h1>Lifecycle Component</h1>
</div>
);
};
export default LifecycleComponent;
State & props
In React.js, state denotes an internal data storage mechanism within a component, reflecting its current data. Accessible via this.state, it's stored individually within components, representing component-specific data. Mutable and updated with setState(), React auto-renders on state changes, fostering dynamic interfaces responsive to user input or data fluctuations. This dynamic management enables components to adjust their output as needed.
On the other hand, props (short for properties) are read-only values that are passed from a parent component to a child component. They are used to provide data or configuration to the child components, allowing them to customize their behavior or appearance based on the provided props.
State and props are both important concepts in React.
JSX
JSX, which stands for JavaScript XML, is a syntax extension for JavaScript. It allows us to write HTML-like code within JavaScript, making it easier to build UI components. JSX offers a familiar and intuitive syntax reminiscent of HTML, making it easily understandable for developers already versed in HTML. This familiarity streamlines the learning curve for adopting JSX.
Moreover, JSX promotes a component-based approach to UI development. Through this methodology, developers can construct reusable UI components, facilitating the creation of intricate user interfaces through composition. One of JSX's notable features is its capability to embed dynamic content, including JavaScript expressions, within the markup. This feature enables the dynamic rendering of UI elements, enhancing user interactivity and experience.
Furthermore, JSX seamlessly integrates with JavaScript, enabling the incorporation of JavaScript expressions and logic directly within JSX code. Additionally, JSX code undergoes static analysis at compile time, providing early detection of errors and potential issues, thus enhancing code reliability and maintainability.
Lastly, JSX code can be optimized for improved performance using tools like Babel, which compile JSX into efficient JavaScript code, ensuring optimal execution speed and resource utilization. Using JSX, we can create React components by combining HTML-like syntax with JavaScript logic. Here's an example of a simple React component written in JSX:
import React, { Component } from 'react';
class WelcomeComponent extends Component {
render() {
return (
<div>
<h1>Hello, World!</h1>
<p>This is a simple React component.</p>
</div>
);
}
}
export default WelcomeComponent;
In the example above, we define a Welcome component that extends the React.Component class. The render method returns JSX code. This is just a basic example, but JSX allows us to create more complex UI components by combining HTML-like tags and JavaScript logic. Below you can see an alternative version.
import React from 'react';
const WelcomeComponent = () => {
return (
<div>
<h1>Hello, World!</h1>
<p>This is a simple React component.</p>
</div>
);
};
export default WelcomeComponent;
This code is an alternative implementation of the WelcomeComponent using a functional component in React. By defining the component as a function instead of a class, we leverage the simplicity and conciseness of functional programming. In this approach, the component returns JSX directly from the function body, making the code more streamlined and easier to read. This modern syntax is widely adopted in React development and offers a more intuitive way of defining components, especially for simpler cases like this one.
Below, I will illustrate the practical implementation of utilizing state within JSX. State, a fundamental concept in React.js, represents the dynamic data managed by a component throughout its lifecycle. Unlike props, which are passed down from parent components, state is managed internally within a component and can be modified over time. This capability enables components to maintain and update their data in response to user interactions, asynchronous events, or other triggers. By leveraging state, developers can create dynamic and interactive user interfaces that respond to changes in data or user input in real-time.
import React, { Component } from 'react';
import ReactDOM from 'react-dom';
class WelcomeComponent extends Component {
constructor(props) {
super(props);
this.state = {
count: 0
};
}
incrementCount = () => {
this.setState({ count: this.state.count + 1 });
};
render() {
return (
<div>
<h1>Hello, World!</h1>
<p>This is a simple React component with state.</p>
<p>Count: {this.state.count}</p>
<button onClick={this.incrementCount}>Increment Count</button>
</div>
);
}
}
export default WelcomeComponent;
Alternative, functional version:
import React, { useState } from 'react';
const WelcomeComponent = () => {
const [count, setCount] = useState(0);
const incrementCount = () => {
setCount(prevCount => prevCount + 1);
};
return (
<div>
<h1>Hello, World!</h1>
<p>This is a simple React component with state.</p>
<p>Count: {count}</p>
<button onClick={incrementCount}>Increment Count</button>
</div>
);
};
export default WelcomeComponent;
Discuss the concept of conditional rendering and how it helps create dynamic UI
Conditional rendering is a powerful concept in web development that allows developers to selectively render components or elements based on certain conditions. It helps create dynamic user interfaces by enabling different parts of the UI to be displayed or hidden based on specific conditions.
With conditional rendering, developers can use conditions like if statements or ternary operators to determine whether a component or element should be rendered or not. This allows for more flexibility in displaying content based on various scenarios.
Conditional rendering is crucial for creating dynamic UI because it enables components to respond to changes in data or user interactions. Conditional rendering allows developers to show or hide components or elements based on specific conditions, such as user input or data changes. This flexibility in rendering helps create interactive and responsive user interfaces.
By using conditional statements, developers can customize the UI based on different scenarios, providing a more personalized experience for users. Conditional rendering also improves performance by reducing unnecessary rendering of components that are not currently needed.
Best Practices in React Development:
When structuring your components, prioritize organization and modularity. Break them into smaller, reusable components whenever feasible to enhance code reusability and facilitate comprehension and maintenance.
Adhere to best practices for state management in your React application. Employ local state for component-specific state and leverage state management libraries like Redux or MobX for managing global state and intricate state interactions.
Embrace immutability when manipulating state and props to ensure predictability and maintain data integrity.
Leverage lifecycle methods such as componentDidMount, componentDidUpdate, and componentWillUnmount to execute specific actions at key points in a component's lifecycle. These actions may encompass data fetching, event subscription or unsubscription, and cleanup tasks.
Boost the performance of your React application by employing optimization techniques such as shouldComponentUpdate or React.memo to prevent unnecessary re-renders. Additionally, utilize React's built-in performance tools like Profiler to identify and address performance bottlenecks effectively. Ensure robust testing coverage for your React components by crafting comprehensive unit tests using frameworks like Jest and Enzyme. This practice fosters reliability and stability in your application.
In addition, make use of React's built-in PropTypes to define the types of props your components expect. This helps catch potential bugs and ensures that your components are used correctly. Another best practice is to optimize the rendering of your components by using techniques like memoization and avoiding unnecessary re-rendering. Finally, make sure to properly handle errors and exceptions in your React application by implementing error boundaries and providing meaningful error messages to users. By following these best practices, you can enhance the quality and performance of your React code.

Provide tips on optimizing performance and handling state effectively.
To enhance performance and manage state efficiently in your React application, you can implement several strategies. Firstly, minimize re-renders by utilizing React's memo or PureComponent to avoid unnecessary updates. Additionally, employ the shouldComponentUpdate lifecycle method to have manual control over when a component should re-render, thus optimizing performance.
Furthermore, optimize performance by avoiding costly operations within render functions. Instead, execute complex calculations or API requests in componentDidMount or componentDidUpdate and store the results in state. Additionally, consider implementing code-splitting and lazy loading techniques to reduce the initial load time of your application, while also ensuring proper memory management to prevent memory leaks and unnecessary state updates, ultimately improving overall performance.
The importance of reusable components and component organization
Reusable components play a pivotal role in software development, offering a multitude of advantages. One significant benefit is efficiency. By employing reusable components, developers can significantly reduce development time and effort. Instead of creating new elements from scratch for each project, they can leverage existing components, streamlining the development process and boosting productivity.
Consistency is another crucial advantage of reusable components. They ensure uniformity in design, functionality, and behaviour across various parts of an application or even multiple applications. This consistency fosters a seamless user experience and diminishes the likelihood of introducing errors or discrepancies, thereby enhancing overall quality.
Moreover, reusable components contribute to scalability. They can be effortlessly adapted and reused in diverse contexts and applications with minimal adjustments. This flexibility simplifies the management of expanding and evolving codebases, enabling developers to maintain pace with changing requirements and technological advancements efficiently.
React vs React Native
React Native is an open-source framework developed by Facebook that allows developers to build mobile applications using the JavaScript programming language. It provides a way to create native mobile apps for iOS and Android platforms using the same codebase. With React Native, mobile developers can write code once and deploy it on multiple platforms, saving time and effort in the development process. It offers a rich set of pre-built components that can be used to create user interfaces, and it also allows for seamless integration with existing native code. React Native combines the benefits of native app performance with the flexibility and efficiency of JavaScript, making it a popular choice for mobile development.
React native is a separate technology from React, and therefore it is understandable that we won't discuss it at this moment. React native is a framework used for building mobile applications, while React is a JavaScript library used for building user interfaces. Although they share some similarities, they have distinct differences in terms of their functionalities and purposes. Thus, if the focus of the discussion is on React and its features, it would be logical to exclude React native from the current conversation.
The future of React.js
The future of React.js looks incredibly promising, as the popular JavaScript library continues to evolve and grow with several exciting developments on the horizon. React has become one of the most widely used front-end frameworks for building user interfaces, thanks to its efficient component-based architecture and virtual DOM rendering.
React Native has surged in popularity for cross-platform mobile app development. This framework enables developers to create high-performing mobile applications using React.js syntax, promoting code-sharing across web and mobile platforms.
React.js may prioritize performance enhancements, including reducing bundle sizes and optimizing rendering with features like Concurrent Mode and Suspense. Given the rising adoption of Jamstack architecture, React.js could refine its support for server-side rendering (SSR) and static site generation (SSG), facilitating the development of faster and SEO-friendly web apps. There's a growing trend towards TypeScript adoption in the React.js ecosystem. React.js might deepen its integration with TypeScript to offer better type safety and developer experience.
Improving developer experience (DX) could involve introducing new tools, APIs, and features like enhanced error handling, debugging tools, and smoother integration with popular developer tools and IDEs. React.js might focus more on accessibility, providing out-of-the-box accessible solutions and advocating for best practices in creating accessible UI components.
Concurrent Mode, initially an experimental feature, could become more prominent, allowing React.js to handle complex UI interactions more efficiently for smoother user experiences. While React's built-in state management is robust, React.js might integrate more closely with popular state management solutions or introduce enhancements to streamline state management. With the rise of web components, React.js might offer better integration and support for interoperability between React components and web components.
React.js could improve support for internationalization (i18n) and localization (l10n) to aid developers in creating multilingual applications targeting global audiences. Innovations in UI/UX could include features or patterns to facilitate the development of more interactive, engaging, and visually appealing user interfaces, aligning with evolving design trends and user expectations.
The React.js community's collaboration, feedback, and contributions will continue to shape the library's development trajectory. It's important to note that these are speculative directions based on the trends and needs observed in the development community. The actual future of React.js will depend on a multitude of factors, including technological advancements, community feedback, and the evolving landscape of web development.
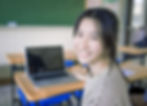
When software development company should use React?
A software development company stands to benefit from considering React.js across various project scenarios, aligning with their unique requirements, team competencies, and overarching goals. React.js emerges as a standout choice particularly suited for Single Page Applications (SPAs), where the entirety of the application operates within the confines of the browser, dynamically updating in response to user interactions. This capability owes much to React.js's adept handling of complex user interfaces, facilitated by its component-based architecture and virtual DOM.
Moreover, for projects demanding immersive, interactive user experiences with dynamic content and intricate state management, React.js equips developers with robust tools to build and sustain such interfaces effectively. Central to React.js's appeal is its advocacy for a component-based architecture, empowering developers to create reusable UI elements. This modularity proves invaluable, especially in the context of large-scale applications necessitating consistent user interface components throughout.
The inherent efficiency of React.js, owing to its virtual DOM and reconciliation algorithm, manifests prominently in scenarios characterized by frequent UI updates, ensuring smooth rendering and seamless user experiences.
In the realm of mobile app development, React Native, leveraging React.js's foundations, presents a compelling solution for crafting cross-platform applications. Its unified codebase for iOS and Android development streamlines the development process, making it an attractive proposition.
Transitioning to React.js may prove seamless for teams versed in JavaScript or experienced with frameworks like Angular or Vue.js, given its conceptual and syntactical similarities. Furthermore, React.js's vibrant ecosystem and thriving community offer a wealth of resources, accelerating development cycles, addressing common challenges, and fostering adherence to best practices.
React.js's backend-agnostic nature facilitates seamless integration with diverse backend technologies, ensuring flexibility and interoperability across different tech stacks. For projects considering the development of Progressive Web Applications (PWAs), React.js emerges as a natural fit, enabling the creation of web applications with native app-like experiences, including offline capabilities and push notifications. The component-based architecture and unidirectional data flow inherent in React.js bolster the maintainability and scalability of applications, a boon for projects anticipating growth and evolution over time.
In conclusion, while React.js presents numerous advantages, the decision to adopt it should stem from a comprehensive evaluation of project requisites, team proficiencies, and long-term aspirations. It's crucial to weigh these factors against the specific needs and constraints of the project to derive maximum benefit from React.js's capabilities.